Create a MySQL User and Grant Privileges
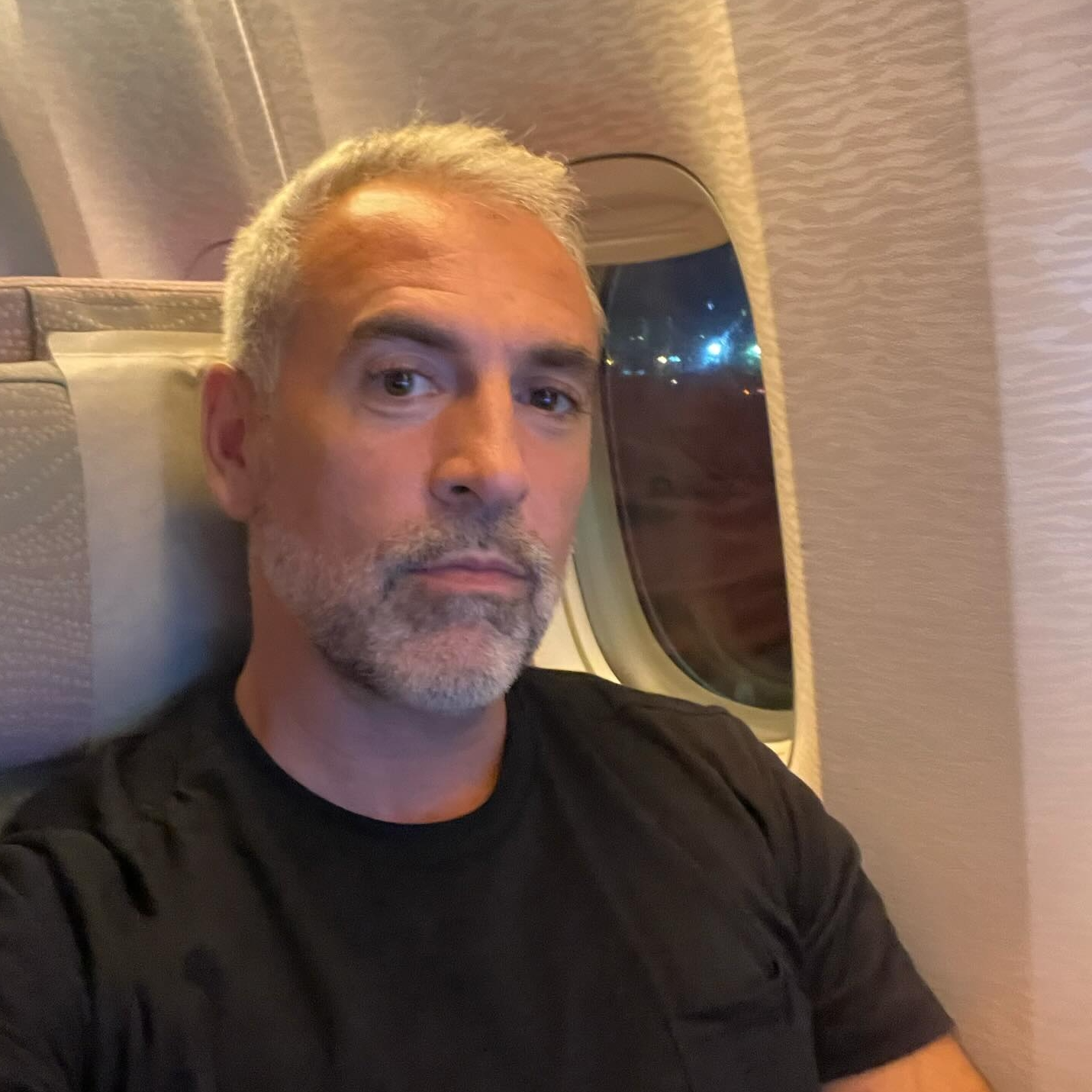
& DevOps Practitioner
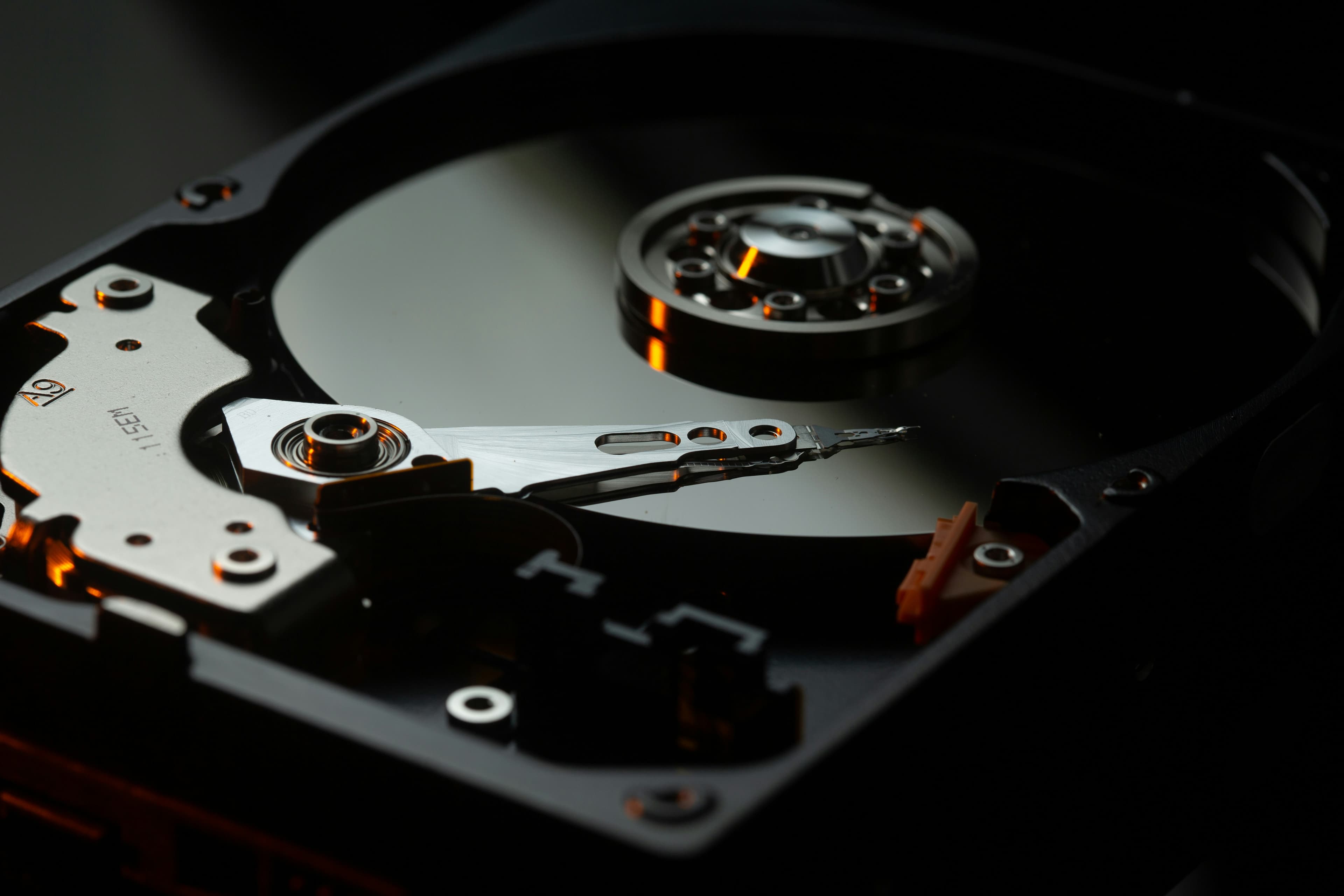
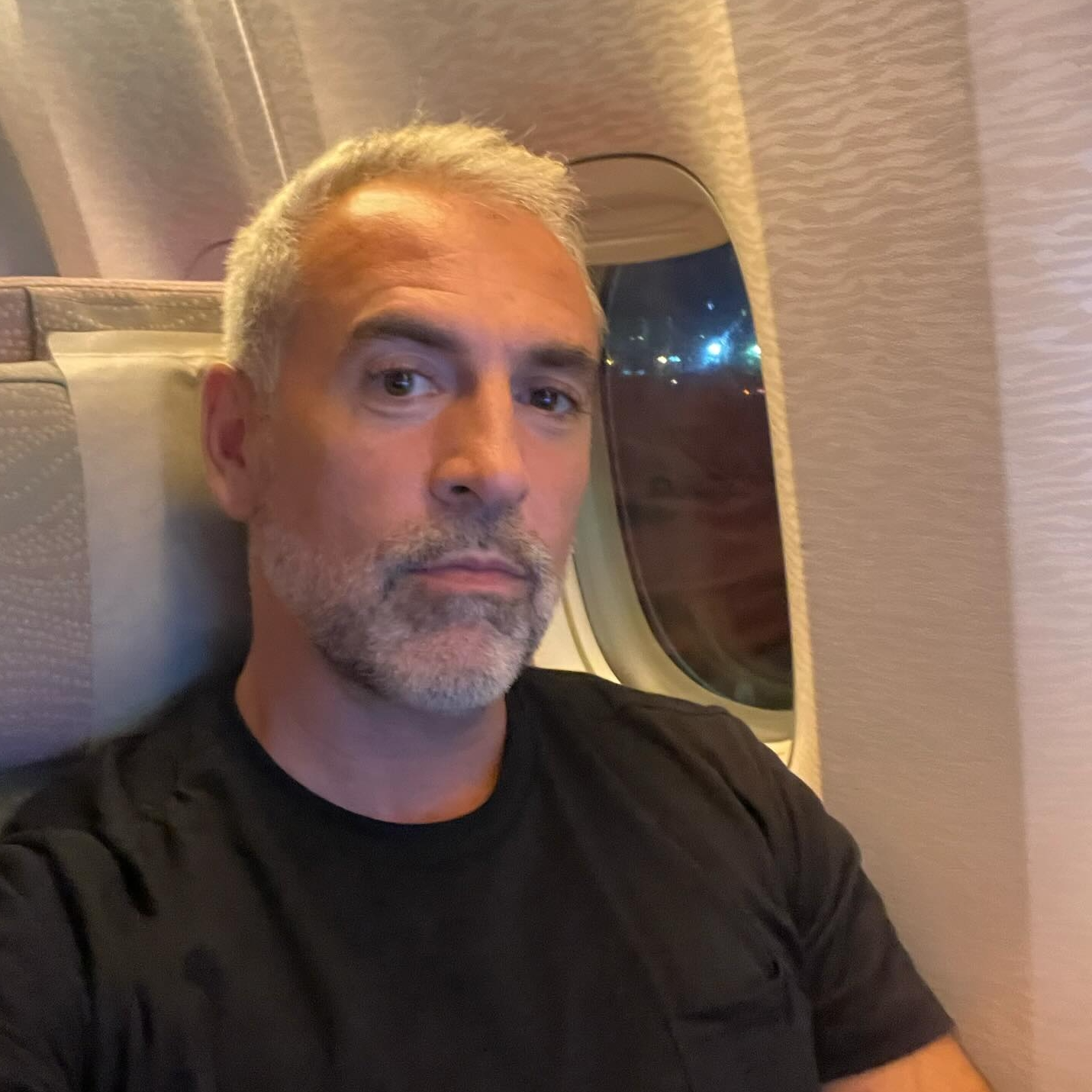
& DevOps Practitioner
When setting up an AWS RDS MySQL database for a backend service, it’s essential to create a dedicated user with the appropriate privileges. This ensures secure and controlled access to your database. Here’s a step-by-step guide to help you achieve this efficiently.
1. Connect to Your RDS Instance
Use your MySQL admin credentials to log in to the database:
mysql -h your-rds-endpoint.rds.amazonaws.com -u admin -p
2. Create a New User
Run the following SQL command to create a new user:
CREATE USER 'your_user'@'%' IDENTIFIED BY 'strongpassword';
This creates a user that can connect from any host (%). However, allowing access from anywhere can pose a security risk. If you know the specific IP address or hostname your application will connect from, it’s better to restrict access to that host:
CREATE USER 'your_user'@'your-app-ip-address' IDENTIFIED BY 'strongpassword';
This improves security by limiting access to only the specified host.
3. Grant Privileges
Next, grant the necessary privileges for the user to access the required database. For example, to grant full access to a specific database:
GRANT ALL PRIVILEGES ON your_database.* TO 'your_user'@'%';
FLUSH PRIVILEGES;
If you only need to grant specific privileges, such as SELECT, INSERT, UPDATE, and DELETE, use:
GRANT SELECT, INSERT, UPDATE, DELETE ON your_database.* TO 'your_user'@'%';
FLUSH PRIVILEGES;
4. Verify the User’s Privileges
After creating the user and granting privileges, it’s important to verify that everything is set up correctly. First, confirm that the user was created successfully:
SELECT user, host FROM mysql.user WHERE user = 'your_user';
Then, check the granted privileges:
SHOW GRANTS FOR 'your_user'@'%';
This will display a list of privileges assigned to the user. For example:
+------------------------------------------------------------------------------------------------------+
| Grants for your_user@% |
+------------------------------------------------------------------------------------------------------+
| GRANT USAGE ON *.* TO `your_user`@`%` |
| GRANT ALL PRIVILEGES ON `your_database`.* TO `your_user`@`%` |
+------------------------------------------------------------------------------------------------------+
5. Correcting Mistakes in Privileges
If you notice that incorrect privileges were granted, you can revoke them using the REVOKE
command. For example, to remove ALL PRIVILEGES
on a specific database:
REVOKE ALL PRIVILEGES ON your_database.* FROM 'your_user'@'%';
FLUSH PRIVILEGES;
To revoke specific privileges, such as DELETE
:
REVOKE DELETE ON your_database.* FROM 'your_user'@'%';
FLUSH PRIVILEGES;
After revoking privileges, verify the changes by running SHOW GRANTS
again:
SHOW GRANTS FOR 'your_user'@'%';
6. Use the Credentials in Your Application
Update your backend service’s configuration with the new database credentials:
DB_HOST=your-rds-endpoint.rds.amazonaws.com
DB_USER=your_user
DB_PASSWORD=strongpassword
DB_NAME=your_database
Now, your application can securely connect to the database using the new user.
Conclusion
By following these steps, you can ensure that your backend service has secure and controlled access to your RDS database. Properly managing users and privileges is crucial for maintaining security and scalability in your infrastructure. Always verify granted privileges and correct any mistakes promptly to avoid unintended access or issues.